A developer's guide to the Google Routes API
How to use the Google Maps Routes API to plan a route with multiple stops, get travel times, avoid tolls and search for places along a route.
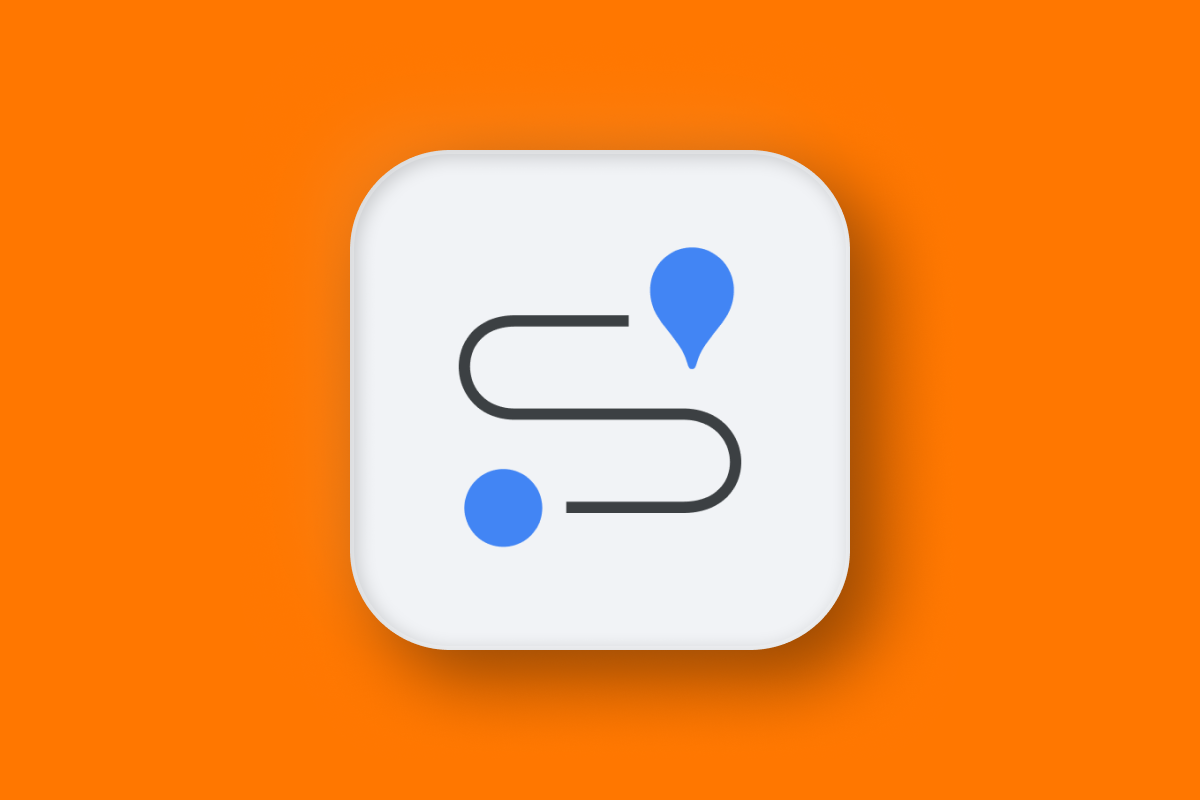
Everyone knows that you can use the Google Maps Routes API to create a route between two points and draw it on a map. But did you know that you can also use it to:
- Calculate travel times and ETAs that account for real time traffic,
- Get turn by turn directions,
- Avoid tolls,
- Plan routes with multiple stops (optimized or not) for
DRIVE
,WALK
,TRANSIT
orBICYCLE
travel modes, - Find the nearest available taxi and,
- Search for points of interest like gas stations, rest stops, or public washrooms along the way?
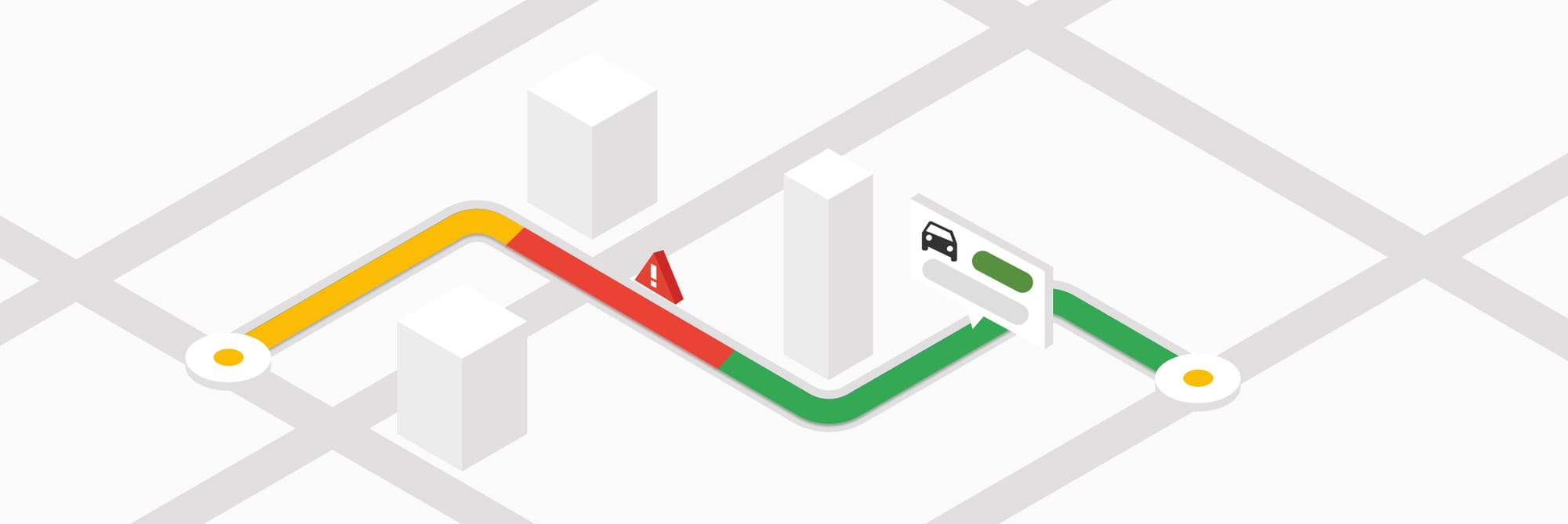
Part 1: A developer's guide to the Google Routes API (this article)
Part 2: Plan a route with multiple stops using the Routes API
Part 3: Using the Google Distance Matrix API for taxi dispatch
Part 4: Google Maps Search Along Route
Part 5: How to draw a route on Google Maps for Android with the Routes API
In this five-part series, I’ll show you how to use three key endpoints: Compute Routes, Compute Route Matrix (from the Routes API), and Search Along Route (part of the Places API). I’ll explore their common use cases, discuss pricing, and end each post with a practical example and working code that you can use in your own projects.
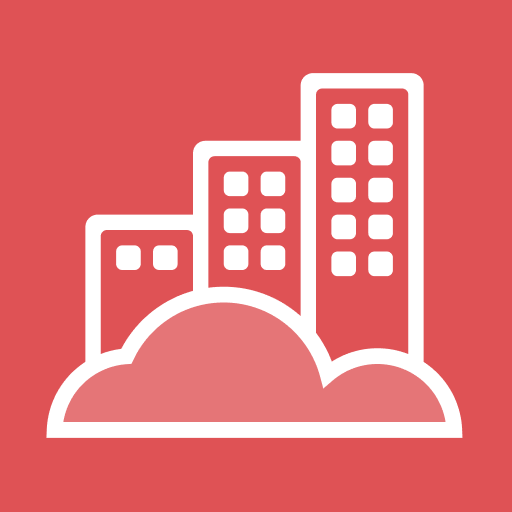
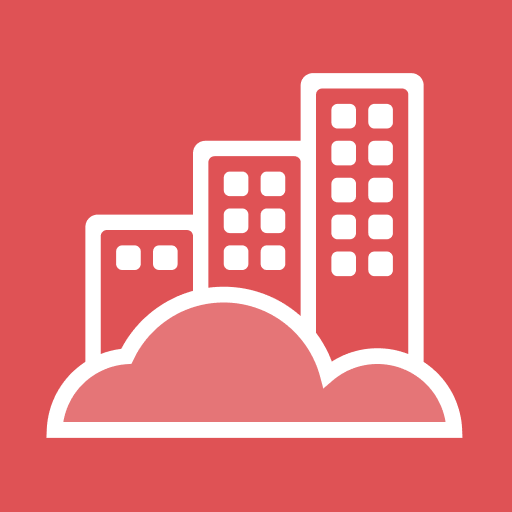
What is the Google Routes API?
The Routes API is the umbrella term for a group of APIs that help you find the fastest way to travel from point A to point B. If you've used the Google Maps App, you're already familiar with what it can do. The Routes API consists of three main endpoints:
- Compute Routes is a multi waypoint routing API that provides estimated travel times (ETAs), driving distances, turn by turn directions and real time traffic conditions for a variety of travel modes. It's used by Google Maps to calculate point to point travel times and distances whenever you use Google Maps for navigation.
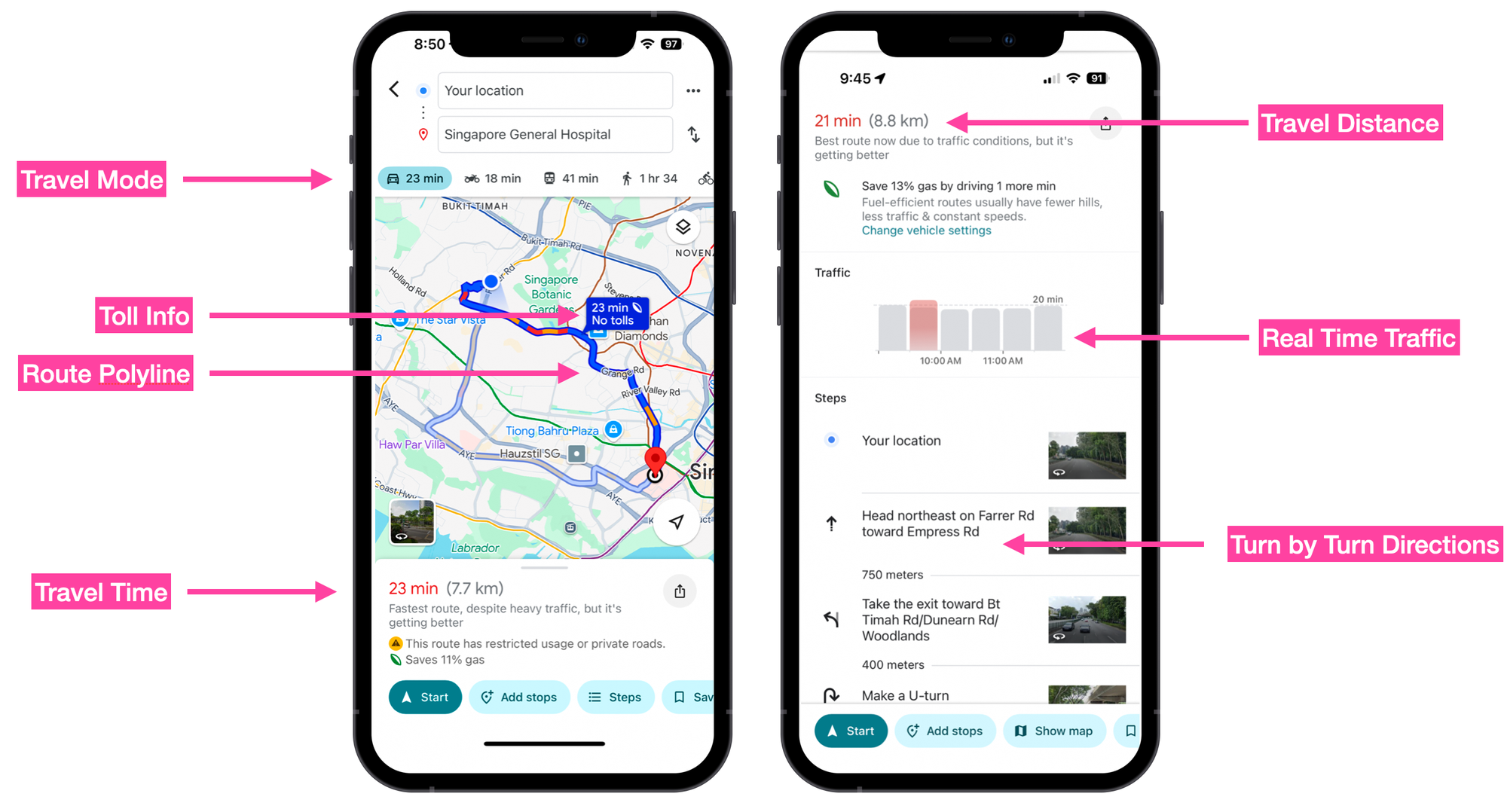
- Compute Route Matrix is a distance matrix API that provides travel distances and durations between multiple origins and destinations. Google Maps uses this API to rank search results based on their distance from your current location.
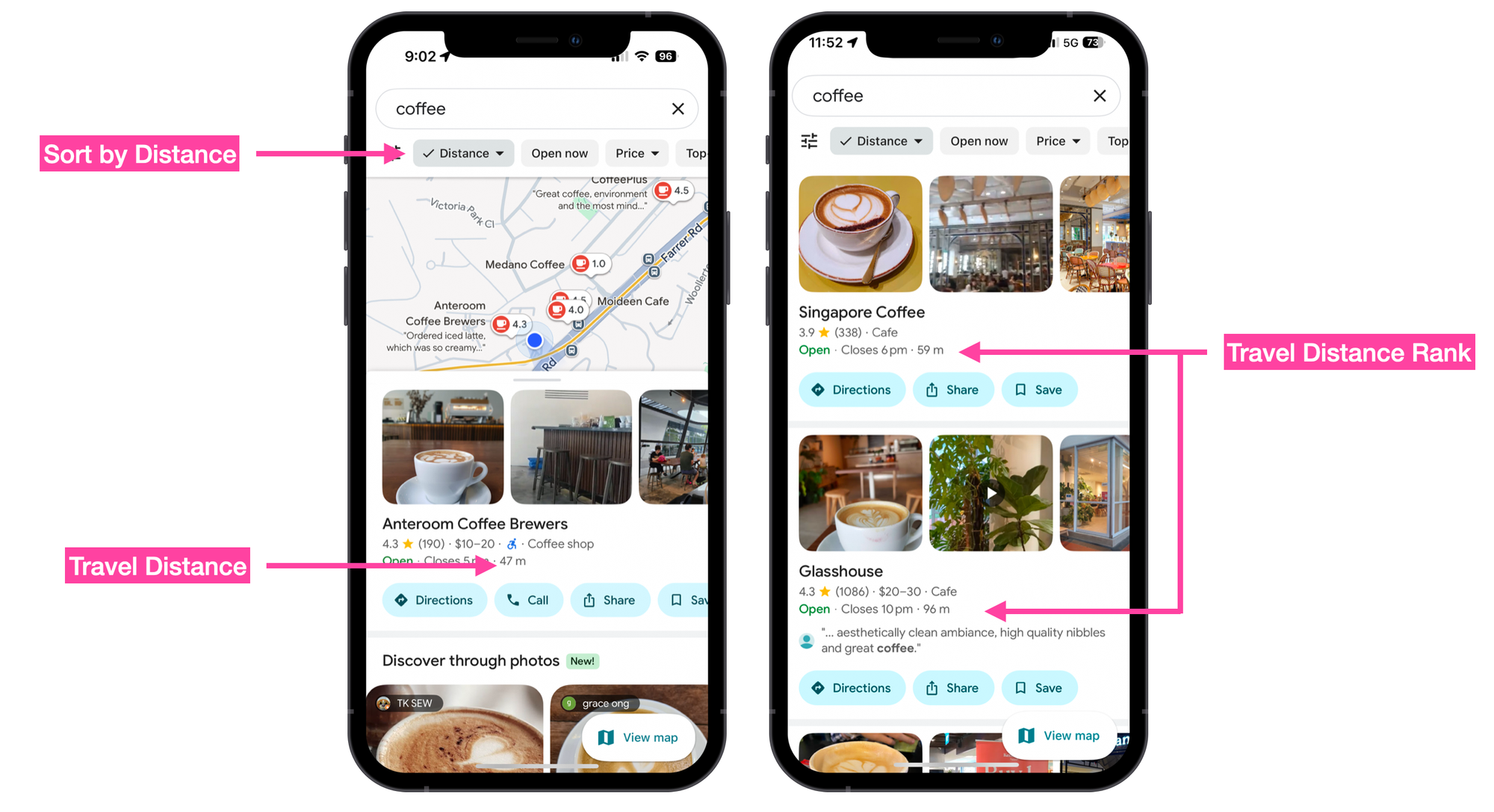
- Search Along Route uses route tokens to perform a Places Text Search, identifying businesses and points of interest located along the route. It's most often used in Car Play when you already have a route programmed into the Google Maps app and need to find a restaurant, coffee shop or rest stop along the way.
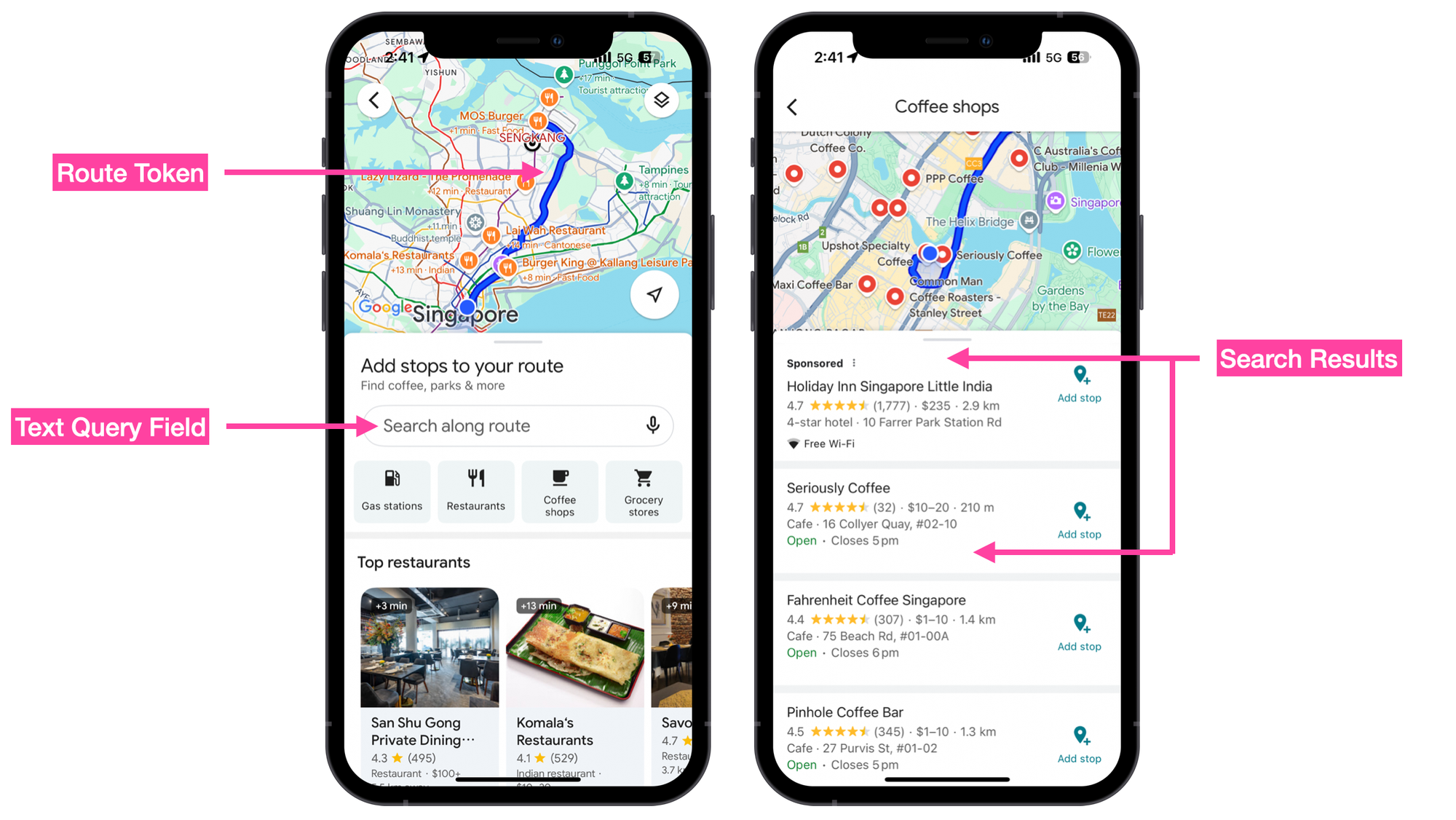
Google Routes API examples
So that's how Google uses the Routes API. Here's what you can do with it.
With the Compute Routes endpoint:
Endpoint POST
https://routes.googleapis.com/directions/v2:computeRoutes
Headers
Content-Type: application/json; charset=utf-8
X-Goog-Api-Key: YOUR_API_KEY
X-Goog-FieldMask: routes.duration,routes.distanceMeters,routes.polyline, routes.legs,routes.travelAdvisory.tollInfo,routes.legs.travelAdvisory.tollInfo,routes.travelAdvisory.speedReadingIntervals
Body
{
"origin": {
"location": {
"latLng": {
"latitude": 49.1951165,
"longitude": -123.1814285
}
}
},
"destination": {
"location": {
"latLng": {
"latitude": 50.1042288,
"longitude": -123.0840866
}
}
},
"travelMode": "DRIVE",
"polylineQuality": "OVERVIEW",
"routingPreference": "TRAFFIC_AWARE",
"departureTime": "2025-10-23T15:00:00Z"
}
Response
Draw a route on a map
The polyline.encodedPolyline
field returned by Compute Routes endpoint can be easily drawn on a Google Map using the <Polyline/>
component or by using a polyline decoder to recover the original latitude and longitude coordinates of each point on the route.
{
"polyline": {
"encodedPolyline": "iggkHlrynVr@j@h@L~C`@b@ERKRYt@iBR{@Hw@FgBHcCRkCt@qNAuAe@mG{BoWW{FEkFDsp@@aTIcHU{Gi@uJESW}CqGqk@gBwPe@oCi@eBi@wAsA_Cq@y@o@m@}Aw@iF_BqBk@}EqAuBs@MQeG_CcAMo@@k@Ns@j@e@l@_@z@aCfJ{AhGS^_@~A}@dDa@z@_@`@gAb@OJuFGMMsDMaIISHGHidAqAwm@{@o\\]uCBeEK{DIq_@e@wGGq_@a@oKKyd@o@ek@s@sJE}HKUQ{JO_AMwAa@s@]"
}
}
Get turn by turn directions to your destination
Need to print out turn by turn directions or display them in an app? Use the navigationInstruction.instructions
field in the steps[]
array returned by Compute Routes.
{
"steps": [
{
"navigationInstruction": {
"maneuver": "DEPART",
"instructions": "Head southwest on Grant McConachie Wy E"
}
}
]
}
Get route distance and travel times adjusted for real time traffic
The Compute Routes endpoint response includes the fields legs.distanceMeters
and legs.duration
, which provide the distance traveled and the travel time for the route. The ETA at your destination can be found by simply adding legs.duration
to the current time.
{
"routes": [
{
"legs": [
{
"distanceMeters": 130429,
"duration": "7812s"
}
]
}
]
}
With the Compute Route Matrix endpoint:
Endpoint POST
https://routes.googleapis.com/directions/v2:computeRoutes
Headers
Content-Type: application/json; charset=utf-8
X-Goog-Api-Key: YOUR_API_KEY
X-Goog-FieldMask: routes.duration,routes.distanceMeters
Body
{
"origins": [
{
"waypoint": {
"location": {
"latLng": {
"latitude": 49.2682989,
"longitude": -123.1830924
}
}
}
}
],
"destinations": [
{
"waypoint": {
"location": {
"latLng": {
"latitude": 49.254563,
"longitude": -123.1121977
}
}
}
},
{
"waypoint": {
"location": {
"latLng": {
"latitude": 49.3137275,
"longitude": -123.0540365
}
}
}
},
{
"waypoint": {
"location": {
"latLng": {
"latitude": 49.2351204,
"longitude": -123.1692661
}
}
}
}
],
"travelMode": "DRIVE",
"routingPreference": "TRAFFIC_AWARE",
"departureTime": "2025-10-23T15:00:00Z"
}
Response
Get distances and travel times to multiple destinations
Rather than calling the Compute Routes endpoint multiple times to obtain distances and travel times for several destinations, Compute Route Matrix provides both distanceMeters
and duration
for multiple destinations in a single API call. This endpoint is commonly used by dispatch algorithms in ride sharing platforms to locate and assign the nearest driver to a passenger's location.
[
{
"destinationIndex": 2,
"status": {},
"distanceMeters": 4827,
"duration": "558s"
},
{
"destinationIndex": 1,
"status": {},
"distanceMeters": 18993,
"duration": "1774s"
}
]
Google Directions API vs Routes API
If you're a long-time Google Maps Platform developer, you might already be using the Directions API to create routes or calculate ETAs. But is it worth upgrading to the Routes API ($8 CPM for the Directions API compared to $10 - $15 CPM for the Routes API)?
My answer is yes, but only if you need the following features.
Real time traffic polylines
The Routes API returns color-coded polylines that dynamically reflect real time traffic conditions. By parsing the travelAdvisory.speedReadingIntervals
in the response, you can provide your users with a visual representation of traffic flow and congestion levels along a route.
{
"speedReadingIntervals": [
{
"endPolylinePointIndex": 13,
"speed": "NORMAL"
},
{
"startPolylinePointIndex": 13,
"endPolylinePointIndex": 15,
"speed": "SLOW"
}
]
}
Two wheeler routing
Places like Jakarta, Manilla and Ho Chi Minh City have huge traffic congestion problems. In these cities, using a motorcycle or scooter mitigates many of these issues because these vehicles can use side streets and bypass congested traffic more easily than cars or larger vehicles. The Routes API lets you specify the travelMode
as "TWO_WHEELER" to account for the unique travel speeds and paths used by two-wheeler vehicles
{
"origin": {
"location": {
"latLng": {
"latitude": 10.8108786,
"longitude": 106.482072
}
}
},
"destination": {
"location": {
"latLng": {
"latitude": 10.4034969,
"longitude": 107.0404779
}
}
},
"travelMode": "TWO_WHEELER",
"polylineQuality": "OVERVIEW",
"departureTime": "2025-10-23T15:00:00Z"
}
Toll data
When providing a route to your users, it's important to consider any toll fees along the way. This is especially true in industries like logistics or last mile delivery, where every cent counts. The travelAdvisory.tollInfo
in the route
object gives you an estimate of what the toll fee in the route will be, expressed in the local currency.
{
"travelAdvisory": {
"tollInfo": {
"estimatedPrice": [
{
"currencyCode": "USD",
"units": "8",
"nanos": 800000000
}
]
}
}
You can even ask the Routes API to return routes that avoid tolls by passing in a avoidTolls
parameter in the request.
{
"routeModifiers": {
"avoidTolls": true,
"avoidHighways": true
}
}
Google Routes API key
To begin using the Google Routes API, you first need to set it up in the Google Cloud Console. Start by creating a new project from the dashboard at https://console.cloud.google.com/ and name the project routes-api-demo
. Click [CREATE].
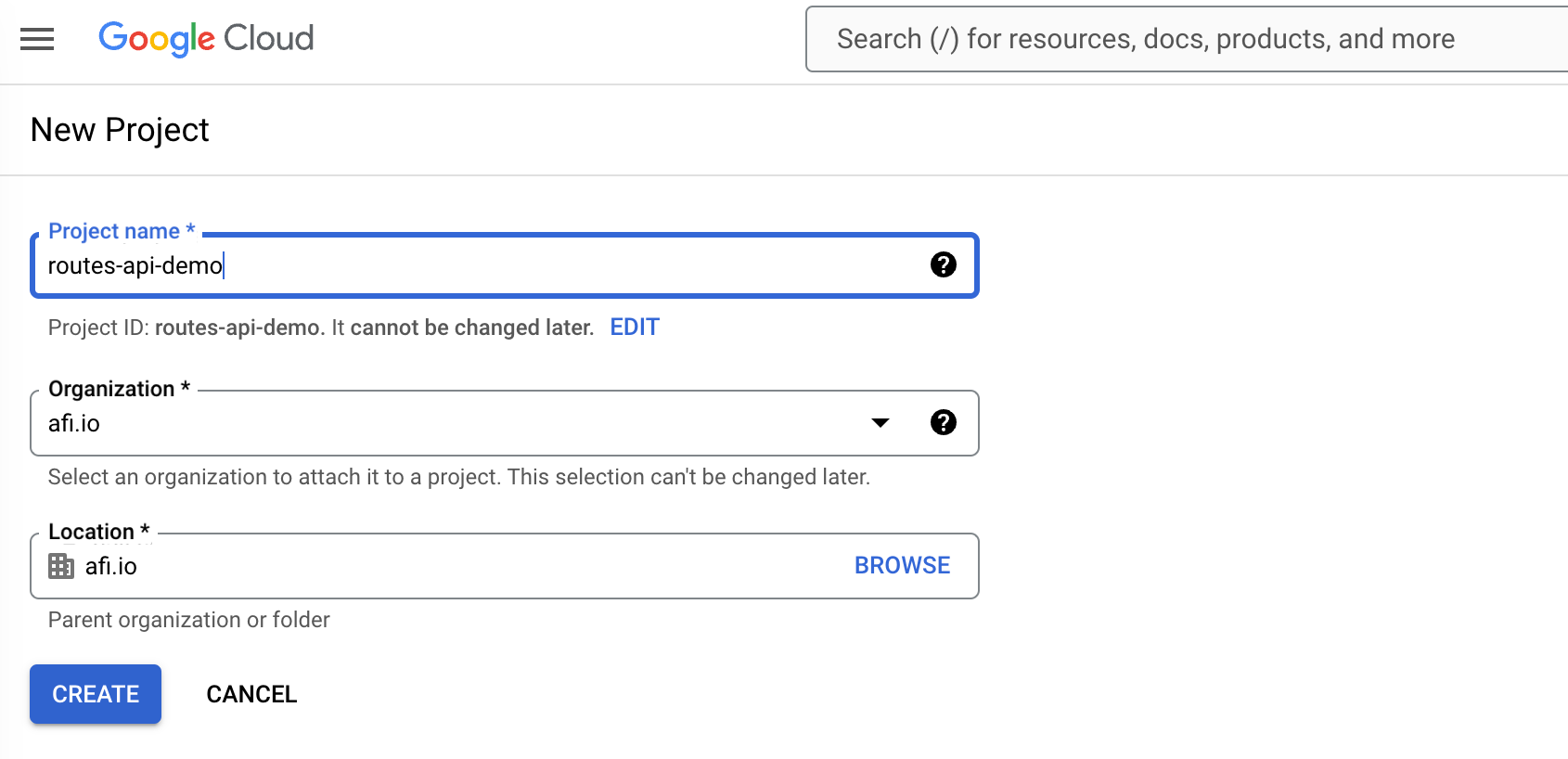
To enable the Google Routes API, navigate to the APIs & Services page by selecting it from the menu on the left. Then, click [+ Enable APIs and Services] and search for "Routes API".
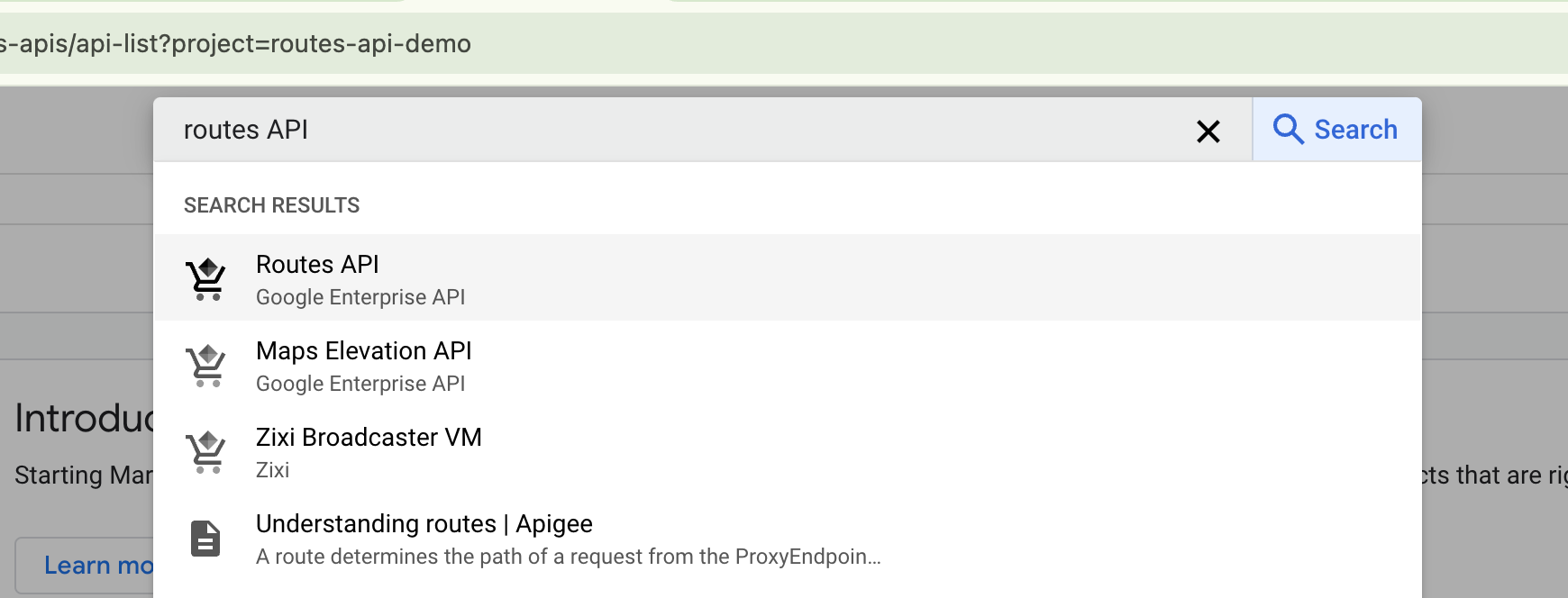
Click on the [Routes API] link and hit the [Enable] button.
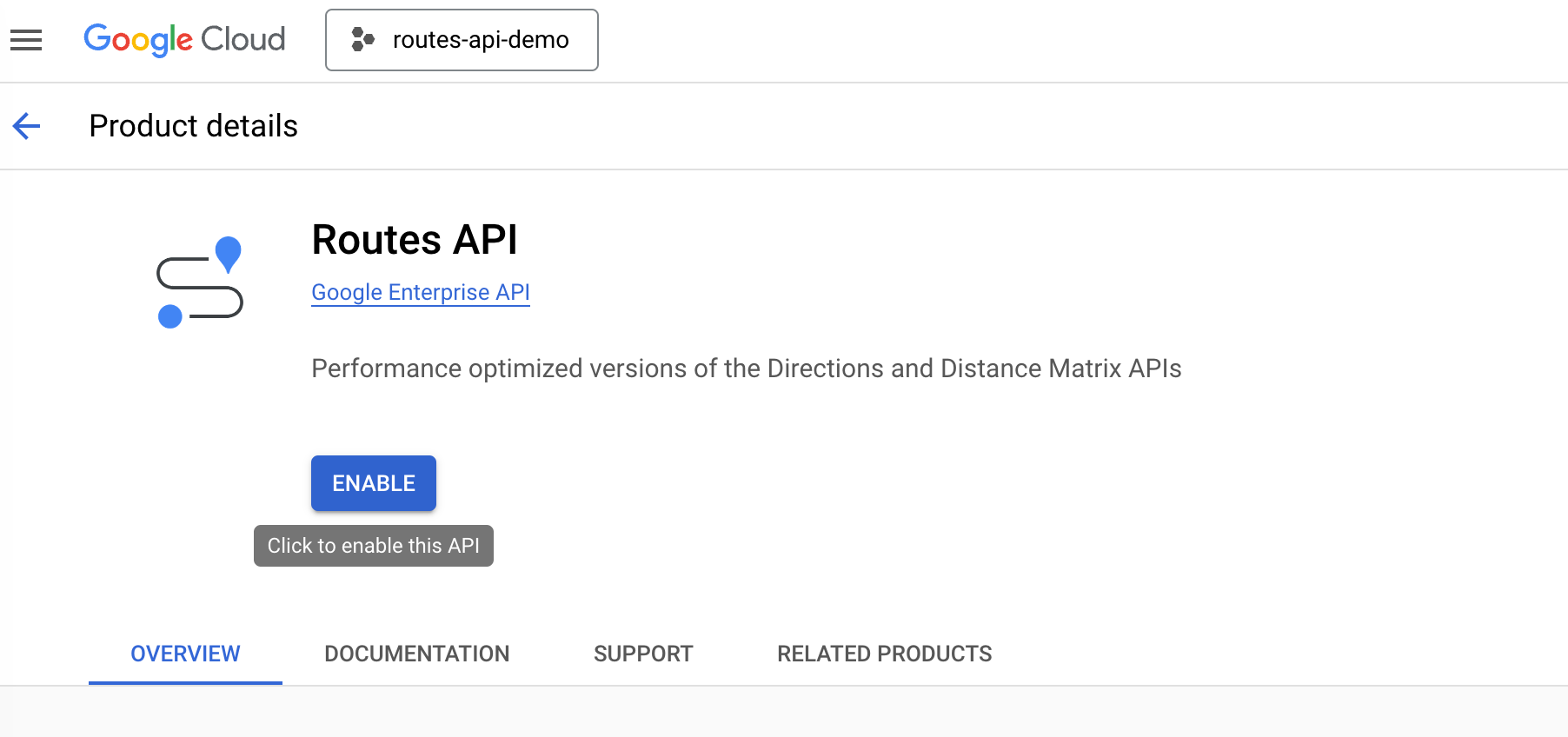
Click [Save]. If you return to the Keys and Credentials page, you can find your new API key by clicking the [Show Key] link on the right.
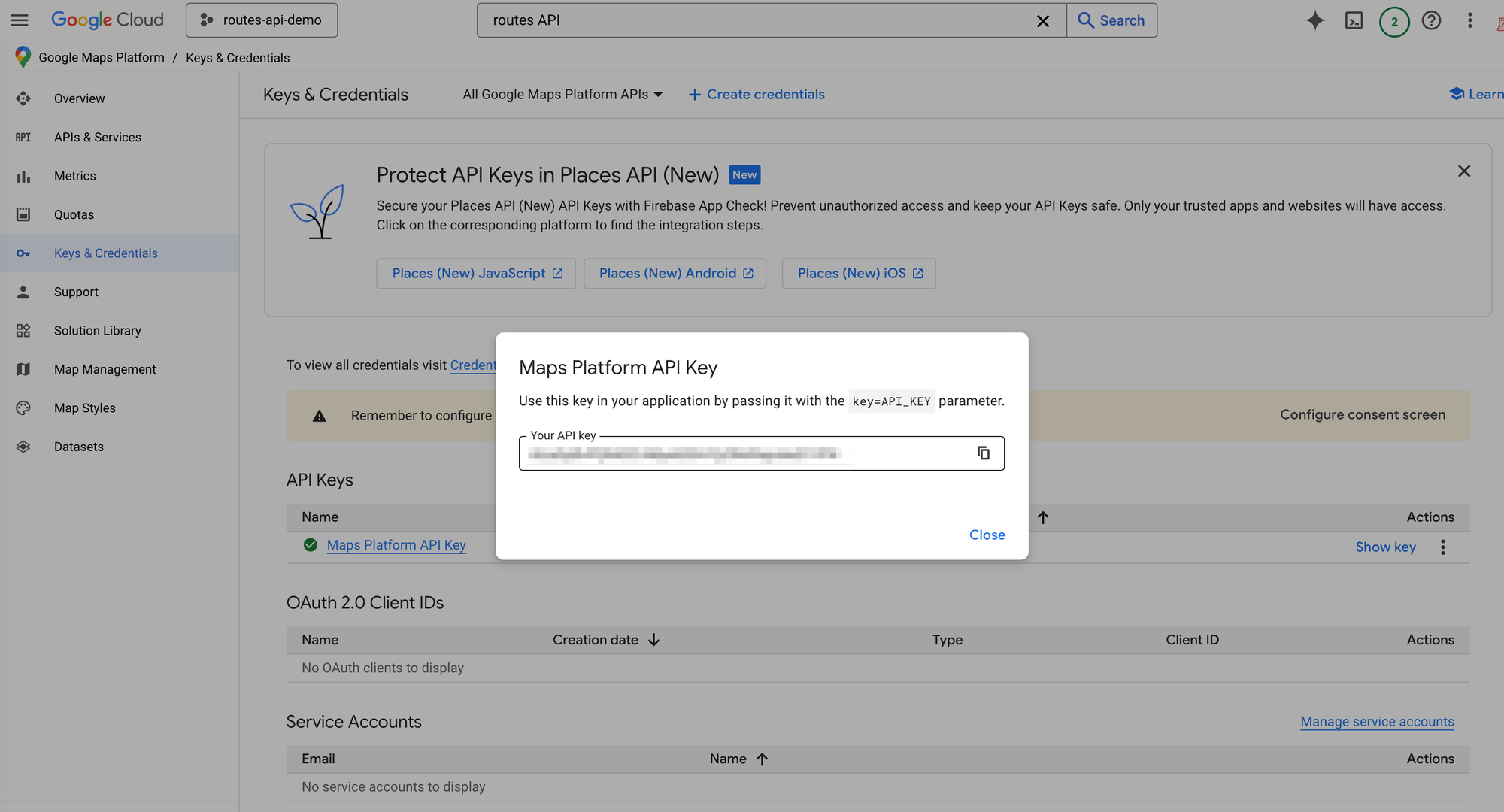
Google Routes API Pricing
Like other Google Maps Platform APIs, the Routes API uses a pay-as-you-go pricing model. There are three pricing tiers for the both the Compute Routes and Compute Route Matrix endpoints - Basic ($5 CPM), Advanced ($10 CPM) and Preferred ($15 CPM). Which pricing tier your API call falls under depends on which fields you included in the X-Goog-FieldMask
header.
Basic: This version of the Routes API gives works just like the Directions API. It provides basic routing information from an origin to a destination, supporting up to 10 intermediate waypoints.
Advanced: The advanced tier includes routing for between 11 and 25 intermediate waypoints, incorporates real time traffic information and allows you to specify Side of the road, Heading and Vehicle stopover modifiers.
Preferred: This highest tier includes everything in the first two tiers plus Two wheeled vehicle routing, Toll calculation and Traffic information on polylines.
For example, if you request for routes.travelAdvisory.tollInfo
by adding it to the X-Goog-FieldMask
header, you are automatically billed at $15 CPM (cost per thousand) for the Routes API (Preferred) SKU.
What's next in this tutorial series
By the end of this course you'll be an expert at integrating advanced routing capabilities into your application. Specifically, I'll teach you how to:
- Draw traffic aware polylines on a Google Map,
- Retrieve trip travel times and distances that take into account real time traffic,
- Use field masks to specify the information you want in the response, ensuring you only access and pay for the data you need,
- Use a distance matrix API to find nearby vehicles,
- Generate a route token to pass to the Google Navigation SDK or Google Text Search API for further computation.
👋 As always, if you have any questions or suggestions for me, please reach out or say hello on LinkedIn.
Part 2: Plan a route with multiple stops using the Routes API